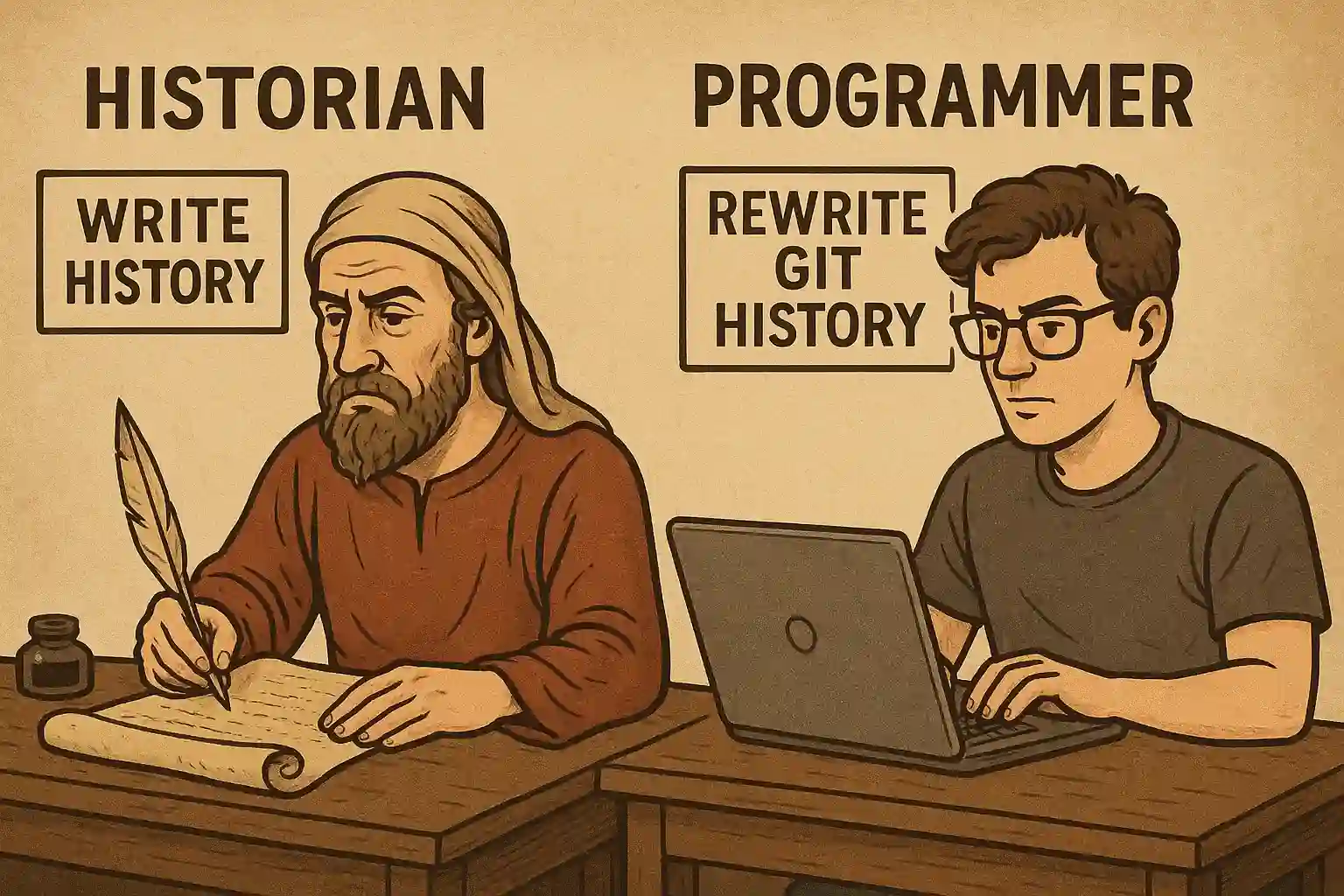
Sometimes you might accidentally commit sensitive files (like .env) or large, unnecessary files (like build artifacts) to your Git repository. In the first case, you expose sensitive data. In the second, your repository becomes bloated and harder to manage.
To completely remove a file from all of Git history, use the following command:
git filter-branch --index-filter 'git rm -rf --cached --ignore-unmatch <path_to_file>' --prune-empty --tag-name-filter cat -- --all
This rewrites your repository history. After running it, you need to force-push the new history to the remote:
git push --force --all
What Happens?
- Local Repository: All affected commits get new hashes.
- Remote Repository: History diverges — you must force push.
- Collaborators: They must re-clone or manually rebase; otherwise, they may retain the sensitive files.
Before:
a1b2c3d (HEAD) Add new feature
e4f5g6h Fix bug
i7j8k9l Initial commit
After:
x9y8z7w (HEAD) Add new feature
p0q1r2s Fix bug
m3n4o5t Initial commit
⚠️ Warning: Others may still have the old data. There’s no guarantee they will re-clone. Use this method at your own risk.
Find and Sort Large Files in Git History
To identify large files still in your Git history, run:
git rev-list --objects --all \
| git cat-file --batch-check='%(objecttype) %(objectname) %(objectsize) %(rest)' \
| awk '/^blob/ {print substr($0,6)}' \
| sort --numeric-sort --key=2 \
| cut --complement --characters=13-40 \
| numfmt --field=2 --to=iec-i --suffix=B --padding=7 --round=nearest
This lists all blobs (files) and their sizes, sorted to help you find the largest ones.